Exploring Date PHP Online: Mastering the Power of Date Handling in PHP
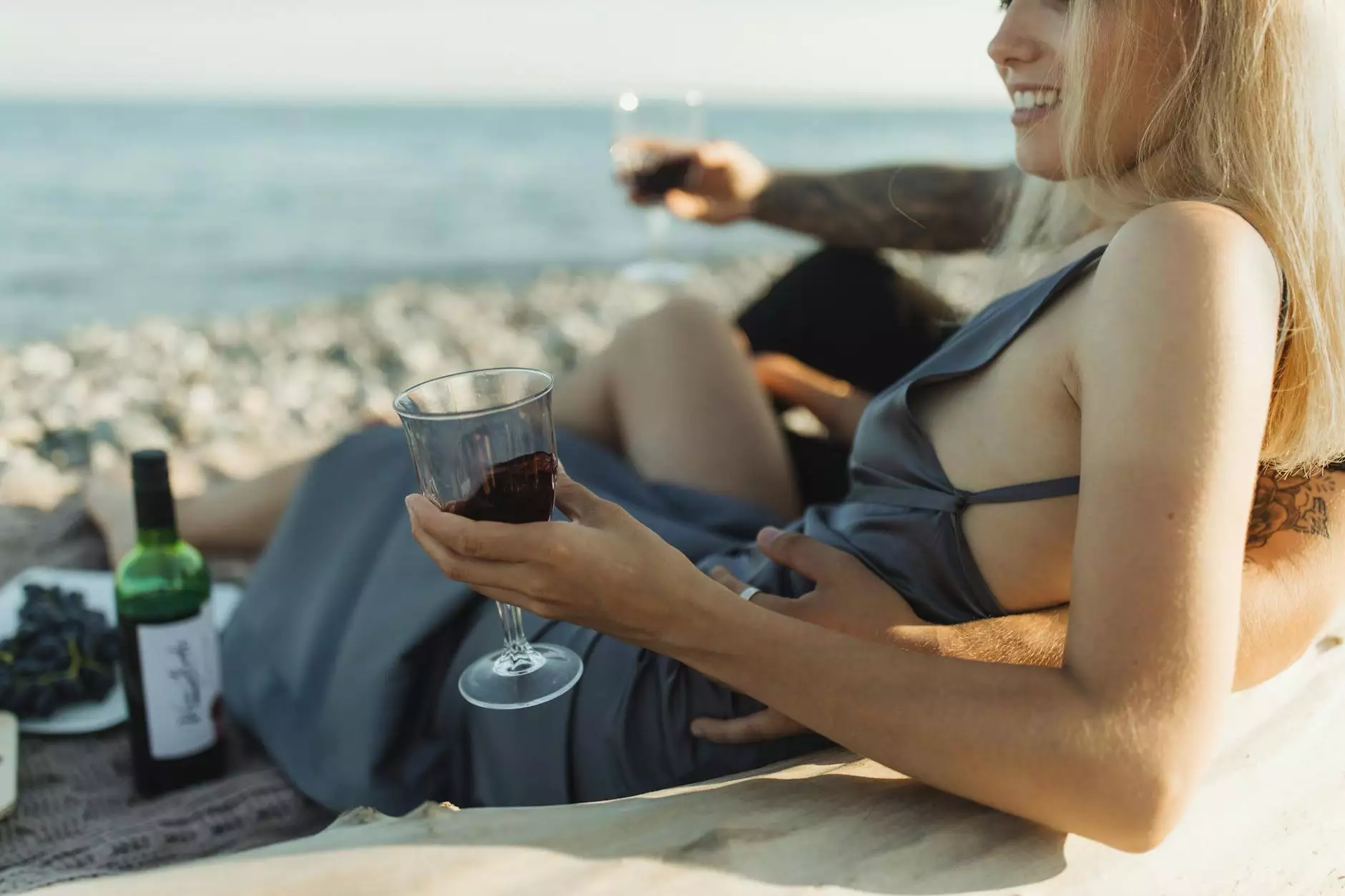
In today’s digital landscape, the significance of date handling in programming cannot be overstated. Businesses, especially those engaged in web design and software development, thrive on their ability to manage and manipulate data effectively. Companies like semalt.tools represent the frontier of innovation in this arena. This article delves deep into date PHP online, spanning its functions, best practices, and application in real-world scenarios.
Understanding the Basics of Dates in PHP
PHP, a widely-used open-source scripting language, provides extensive features for dealing with dates and times. Understanding how to leverage the date functions in PHP is essential for any developer aiming to deliver robust applications.
The Importance of Date Handling
Effective date handling is crucial for various reasons:
- Data Accuracy: Accurate date records are essential for maintaining the integrity of databases.
- User Experience: Users expect timely and relevant information presented in a user-friendly manner.
- Business Operations: Many business operations depend on dates—think transactions, reports, and more.
Key Functions for Date PHP Online
The PHP language offers a variety of built-in functions to facilitate date handling. Let’s explore some of the core functions that you can leverage:
1. date() Function
The date() function formats a local date and time, making it a powerful tool in your PHP toolbox. Here’s how it works:
echo date('Y-m-d H:i:s'); // Outputs: 2023-10-03 14:20:00This function can format the date in various ways by altering the string passed into it. For instance:
- date('d/m/Y') will output the date in the format of '03/10/2023'.
- date('l') returns the day of the week.
2. strtotime() Function
The strtotime() function is used to convert a date string into a Unix timestamp. This can be particularly useful for calculations. Here’s an example:
echo strtotime('now'); // Outputs the current timestamp3. DateTime Class
The DateTime class in PHP provides a more flexible and object-oriented approach to date and time handling:
$date = new DateTime('2023-10-03'); echo $date->format('Y-m-d H:i:s');Practical Applications of Date PHP Online
Now that we understand the functions, let’s look at practical applications where date PHP online plays a pivotal role:
1. Creating Blogs and Timelines
In the development of blogs or event timelines, displaying the correct date and time is crucial. By utilizing the aforementioned functions, you can dynamically generate posts with accurate timestamps.
2. E-commerce Platforms
E-commerce sites thrive on effective date management. For instance, displaying sale dates, shipping timelines, and transaction histories can be streamlined using PHP's date functions.
3. Scheduling Systems
Applications that involve appointments or bookings require precise date handling capabilities. Utilizing the DateTime class allows developers to manage complex scheduling easily.
Strategies for Optimizing Date Handling in PHP
To maximize the effectiveness of date handling in your applications, consider the following strategies:
1. Use Consistent Date Formatting
Maintaining a consistent date format throughout your application ensures better readability and reduces errors. It also aids in debugging when something goes wrong.
2. Validate User Input
When users input dates, it's critical to validate their input to avoid unexpected behavior in your application. Use checkdate() to ensure that the entered date is valid.
3. Leverage Time Zones
Many applications serve users across various time zones. PHP allows you to set a default time zone using:
date_default_timezone_set('America/New_York');Advanced Date Manipulation Techniques
Once you have mastered the basics, you might want to explore more advanced techniques:
1. Date Calculations
Calculating date intervals can be done easily using the DateInterval class:
$date = new DateTime('2023-10-03'); $date->add(new DateInterval('P1D')); // Add one day echo $date->format('Y-m-d'); // Outputs: 2023-10-042. Comparing Dates
To compare two dates, you can use comparison operators directly with DateTime objects:
$date1 = new DateTime('2023-10-03'); $date2 = new DateTime('2023-10-04'); if ($date1